How to Build a Text To Speech in Bootstrap, TailwindCSS, JSON & JavaScript
Sunday, September 08, 2024
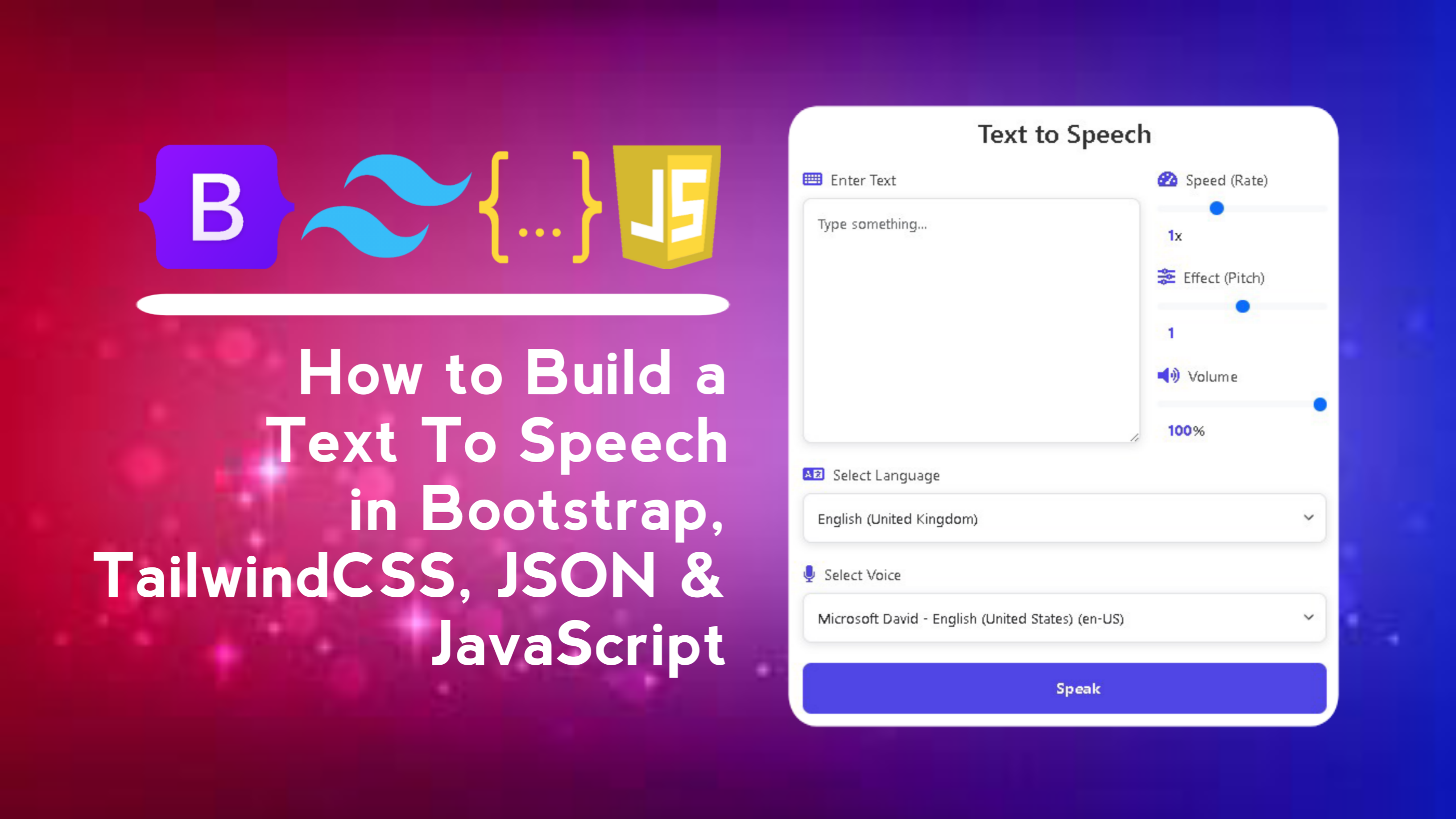
Text To Speech (TTS) is a technology that can convert written text into spoken sound. This technology allows computers or other digital devices to "read" the given text using a synthetic voice that resembles the human voice. TTS is very useful for a variety of applications, especially for accessibility and communication needs.
In this blog post, I will walk you through creating an interactive Text to Speech (TTS) application using Bootstrap, TailwindCSS, JSON, and JavaScript. We will replicate the main features of TTS such as text input, speed control, pitch, volume, language selection, and voice selection options. The application will also be responsive and have an attractive interface, leveraging a combination of two popular CSS frameworks and the dynamic functionality of JavaScript. Let’s get started building a project that allows text to be converted to speech in real-time!
As you work on this project, you’ll learn how to structure and style websites effectively to ensure they look great on all devices.
Key Features:
- Dynamic Text Input: Users can type text to be converted into voice with a simple and intuitive interface.
- Rate Control: Adjust the speech rate with the slider to increase or decrease the tempo of the resulting voice.
- Pitch Adjustment: Change the pitch of the sound via the pitch controller, allowing for variations in the pitch of the sound according to preference.
- Volume Settings: Adjust the volume level of the sound produced with easy-to-use controls.
- Language Selection: Support for multiple languages, providing flexibility in text to speech conversion in various dialects.
- Voice Selection: Users can select a voice from a variety of options, both male and female, depending on their preference.
- Responsive Interface: Mobile-friendly and responsive design using a combination of Bootstrap and TailwindCSS.
- Modern Theme: Combines visual elements from Bootstrap and Tailwind to create a clean and modern interface.
- JSON Support for Language Data: Use JSON to map and manage supported languages in an application.
- Accessibility: Enables users with visual impairments to convert text to speech, supporting better accessibility on the web.
With these features, your Text To Speech application will have complete functionality and an attractive design, making it not only useful but also fun to use.
Why Build a Text To Speech in Bootstrap, TailwindCSS, JSON & JavaScript?
- Bootstrap and TailwindCSS: Master how to use two popular CSS frameworks to build modern, responsive user interfaces.
- Interacting with the Web Speech API: Learn how to use the JavaScript API to convert text to speech and control speed, pitch, volume, and select multiple languages.
- JSON Data Management: Use JSON files to store and manage voice and language data in your app.
- Dynamic Functionality with JavaScript: Understand how to implement dynamic features such as sound selection, slider controls, and action buttons using JavaScript.
- Web Accessibility Improvements: Create accessibility-enabled applications with TTS, making them more inclusive for users with visual impairments.
By building this Text to Speech project using Bootstrap, TailwindCSS, JSON, and JavaScript, you can gain the following skills:
This project will provide a solid foundation in responsive web design and front-end development, paving the way for more complex web projects in the future. Good luck and may the skills you gain be useful in developing future projects!
Steps to build a Text To Speech in Bootstrap, TailwindCSS, JSON & JavaScript
Here are the steps to create a Text-To-Speech application using Bootstrap, TailwindCSS, JSON and JavaScript.
1. Set Up HTML Structure
Use the HTML structure provided. Here's a simplified overview:
<!DOCTYPE html>
<!-- Coding By CodingIndo - https://codingindo.vercel.app/ -->
<html lang="en">
<head>
<!-- Meta tag for character encoding -->
<meta charset="UTF-8">
<!-- Meta tag to make the viewport responsive on mobile devices -->
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Page title -->
<title>Text To Speech</title>
<!-- Link to Bootstrap CSS file -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css" rel="stylesheet">
<!-- Link to Tailwind CSS JavaScript file -->
<script src="https://cdn.tailwindcss.com"></script>
<!-- Link to Font Awesome CSS file -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0-beta3/css/all.min.css" />
<style>
/* Styling for the page body */
body {
background: linear-gradient(135deg, #667eea 0%, #764ba2 100%);
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
}
/* Styling for card */
.card {
background-color: #ffffff;
border-radius: 15px;
box-shadow: 0 12px 28px rgba(0, 0, 0, 0.2);
padding: 2.5rem;
max-width: 800px;
margin: auto;
}
/* Styling for card title */
.card h2 {
color: #333;
}
/* Styling for form controls and form select */
.form-control, .form-select {
border-radius: 10px;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1);
}
/* Styling for input group text */
.input-group-text {
background-color: #f3f4f6;
border-radius: 10px;
}
/* Styling for primary button */
.btn-primary {
background-color: #4f46e5;
border: none;
border-radius: 10px;
padding: 0.75rem 1rem;
transition: background-color 0.3s ease, transform 0.2s;
}
/* Styling for hover on primary button */
.btn-primary:hover {
background-color: #4338ca;
transform: scale(1.05);
}
/* Styling for labels */
label {
font-size: 1.125rem;
color: #444;
}
/* Styling for input range */
input[type="range"] {
accent-color: #4f46e5;
}
/* Styling for display values */
.value-display {
display: inline-block;
font-weight: bold;
font-size: 1.125rem;
color: #4f46e5;
margin-left: 10px;
}
/* Styling for icon */
.icon {
color: #4f46e5;
font-size: 1.25rem;
margin-right: 10px;
}
</style>
</head>
<body>
<!-- Container for cards -->
<div class="card p-6 shadow-lg">
<!-- Card title -->
<h2 class="text-3xl font-semibold text-center mb-4">Text to Speech</h2>
<!-- Form for text input and other settings -->
<div class="d-flex justify-content-between align-items-start">
<!-- Form group for text input -->
<div class="form-group mb-4" style="flex: 1; margin-right: 20px;">
<label for="textInput" class="form-label"><i class="fas fa-keyboard icon"></i>Enter Text</label>
<textarea class="form-control p-3" id="textInput" rows="4" placeholder="Type something..."></textarea>
</div>
<!-- Form group for rate, pitch, and volume settings -->
<div style="flex: 0.5;">
<div class="form-group mb-4">
<label for="rateRange" class="form-label"><i class="fas fa-tachometer-alt icon"></i>Speed (Rate)</label>
<input type="range" id="rateRange" class="form-range" min="0.5" max="2" step="0.1" value="1">
<span class="value-display" id="rateValue">1</span>x
</div>
<div class="form-group mb-4">
<label for="pitchRange" class="form-label"><i class="fas fa-sliders-h icon"></i>Effect (Pitch)</label>
<input type="range" id="pitchRange" class="form-range" min="0" max="2" step="0.1" value="1">
<span class="value-display" id="pitchValue">1</span>
</div>
<div class="form-group mb-4">
<label for="volumeRange" class="form-label"><i class="fas fa-volume-up icon"></i>Volume</label>
<input type="range" id="volumeRange" class="form-range" min="0" max="1" step="0.1" value="1">
<span class="value-display" id="volumeValue">100</span>%
</div>
</div>
</div>
<!-- Form group to select language -->
<div class="form-group mb-4">
<label for="languageSelect" class="form-label"><i class="fas fa-language icon"></i>Select Language</label>
<select id="languageSelect" class="form-select p-3"></select>
</div>
<!-- Form group to select sound -->
<div class="form-group mb-4">
<label for="voiceSelect" class="form-label"><i class="fas fa-microphone icon"></i>Select Voice</label>
<select id="voiceSelect" class="form-select p-3"></select>
</div>
<!-- Button to start text to speech -->
<button id="speakBtn" class="btn btn-primary w-full py-3 text-lg font-bold"><i class="fas fa-play icon"></i>Speak</button>
</div>
<!-- Link to JavaScript file for Text To Speech -->
<script src="script.js"></script>
<!-- Link to Bootstrap JavaScript file -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
Explanation
Number | Line of Code | Explanation |
---|
2. Create JSON File
Create a file named language.json with the following structure.
{
"af": "Afrikaans (Suid-Afrika)",
"sq": "Shqip (Shqipëri)",
"am": "አማርኛ (ኢትዮጵያ)",
"ar": "العربية (السعودية)",
"az": "Azərbaycan (Azərbaycan)",
"bn": "বাংলা (বাংলাদেশ)",
"bs": "Bosanski (Bosna i Hercegovina)",
"bg": "Български (България)",
"my": "ဗမာ (မြန်မာ)",
"ca": "Català (Andorra)",
"zh": "中文(中国)",
"hr": "Hrvatski (Hrvatska)",
"cs": "Čeština (Česká Republika)",
"da": "Dansk (Danmark)",
"nl": "Nederlands (Nederland)",
"en": "English (United Kingdom)",
"et": "Eesti (Estonia)",
"fil": "Tagalog (Pilipinas)",
"fi": "Suomen (Suomi)",
"fr": "Français (France)",
"gl": "Galego (España)",
"ka": "ქართული (საქართველო)",
"de": "Deutsch (Deutschland)",
"el": "Ελληνικά (Ελλάδα)",
"gu": "ગુજરાતી (ભારત)",
"he": "עברית (ישראל)",
"hi": "हिन्दी (भारत)",
"hu": "Magyar (Magyarország)",
"is": "Íslenska (Ísland)",
"id": "Bahasa Indonesia (Indonesia)",
"ga": "Gaeilge (Éire)",
"it": "Italiano (Italia)",
"ja": "日本語 (日本)",
"jv": "Basa Jawa (Indonesia)",
"kn": "ಕನ್ನಡ (ಕೆನಡಾ)",
"kk": "Қазақ (Қазақстан)",
"km": "ខ្មែរ (កម្ពុជា)",
"ko": "한국어 (대한민국)",
"lo": "ລາວ (ປະເທດລາວ)",
"lv": "Latviešu (Latvija)",
"lt": "Lietuvių (Lietuva)",
"mk": "Македонски (Северна Македонија)",
"ms": "Bahasa Melayu (Malaysia)",
"ml": "മലയാളം (ഇന്ത്യ)",
"mt": "Malti (Malta)",
"mr": "मराठी (भारत)",
"mn": "Монгол (Монгол)",
"ne": "नेपाली (नेपाल)",
"nb": "Norsk (Norge)",
"ps": "پښتو (افغانستان)",
"fa": "فارسی (ایران)",
"pl": "Polski (Polska)",
"pt": "Português (Portugal)",
"ro": "Română (România)",
"ru": "Русский (Россия)",
"sr": "Српски (Србија)",
"si": "සිංහල (ශ්රී ලංකා)",
"sk": "Slovenčina (Slovensko)",
"sl": "Slovenščina (Slovenija)",
"so": "Soomaali (Soomaaliya)",
"es": "Español (España)",
"su": "Basa Sunda (Indonésia)",
"sw": "Kiswahili (Kenya)",
"sv": "Svenska (Sverige)",
"ta": "தமிழ் (சிங்கப்பூர்)",
"te": "తెలుగు (భారతదేశం)",
"th": "ไทย (ประเทศไทย)",
"tr": "Türkçe (Türkiye)",
"uk": "Українська (Україна)",
"ur": "اردو (پاکستان)",
"uz": "O'zbekcha (O'zbekiston)",
"vi": "Tiếng Việt (Việt Nam)",
"cy": "Cymraeg (Cymru)",
"zu": "IsiZulu (Ningizimu Afrika)"
}
Explanation
Language Code | Language Name (Country) | Explanation |
---|
3. Add JavaScript Logic
Create a file named script.js to handle the functionality:
// Get text input element from DOM
const textInput = document.getElementById('textInput');
// Get the language select element from the DOM
const languageSelect = document.getElementById('languageSelect');
// Get the sound select element from the DOM
const voiceSelect = document.getElementById('voiceSelect');
// Get the talk button element from the DOM
const speakBtn = document.getElementById('speakBtn');
// Get the speed range element from the DOM
const rateRange = document.getElementById('rateRange');
// Get the tone range element from the DOM
const pitchRange = document.getElementById('pitchRange');
// Get volume range elements from DOM
const volumeRange = document.getElementById('volumeRange');
// Get the speed value element from the DOM
const rateValue = document.getElementById('rateValue');
// Get the tone value element from the DOM
const pitchValue = document.getElementById('pitchValue');
// Get the volume value element from the DOM
const volumeValue = document.getElementById('volumeValue');
// Initialize an array to store sounds
let voices = [];
// Function to load language names from JSON file
function loadLanguageNames() {
return fetch('language.json')
.then(response => response.json())
.then(data => data);
}
// Function to fill the sound select element with available sounds.
function populateVoices() {
voices = speechSynthesis.getVoices();
voiceSelect.innerHTML = '';
const selectedLang = languageSelect.value;
voices
.filter(voice => voice.lang.startsWith(selectedLang))
.forEach(voice => {
const option = document.createElement('option');
option.textContent = `${voice.name} (${voice.lang})`;
option.value = voice.name;
voiceSelect.appendChild(option);
});
}
// Function to fill the language select element with available languages.
function populateLanguages(languageNames) {
const uniqueLangs = [...new Set(voices.map(voice => voice.lang.split('-')[0]))];
languageSelect.innerHTML = '';
uniqueLangs.forEach(lang => {
const option = document.createElement('option');
option.value = lang;
option.textContent = languageNames[lang] || lang;
languageSelect.appendChild(option);
});
populateVoices();
}
// Add event listener to voice when language changes
languageSelect.addEventListener('change', populateVoices);
// Add event listener to update the speed value when the input changes.
rateRange.addEventListener('input', () => {
rateValue.textContent = rateRange.value;
});
// Add event listener to update pitch value when input changes
pitchRange.addEventListener('input', () => {
pitchValue.textContent = pitchRange.value;
});
// Add an event listener to update the volume value when the input changes.
volumeRange.addEventListener('input', () => {
volumeValue.textContent = Math.round(volumeRange.value * 100);
});
// Function to start text to speech
function speakText() {
const text = textInput.value;
if (!text) return;
const utterance = new SpeechSynthesisUtterance(text);
const selectedVoiceName = voiceSelect.value;
const selectedVoice = voices.find(voice => voice.name === selectedVoiceName);
utterance.voice = selectedVoice;
utterance.rate = rateRange.value;
utterance.pitch = pitchRange.value;
utterance.volume = volumeRange.value;
speechSynthesis.speak(utterance);
}
// Add event listener to load voice and language when voice changes
speechSynthesis.onvoiceschanged = () => {
loadLanguageNames().then(languageNames => {
voices = speechSynthesis.getVoices();
populateLanguages(languageNames);
});
};
// Add event listener for speak button
speakBtn.addEventListener('click', speakText);
Explanation
Number | Line of Code | Explanation |
---|
- Open your index.html file in a web browser.
- Test the input, sliders, and language/voice selection functionality.
- Make sure the text-to-speech feature works as expected.
4. Run Your Project
- Cross-Browser Compatibility: Ensure that your Text to Speech application works across different web browsers (e.g., Chrome, Firefox, Safari) and devices. Testing on multiple platforms will help you identify and fix compatibility issues.
- Performance Optimization: Optimize the performance of your application to handle large texts or frequent requests efficiently. This includes minimizing the use of heavy libraries and optimizing JavaScript functions.
- Error Handling: Implement robust error handling to manage scenarios where the speech synthesis may fail, such as unsupported languages or issues with the SpeechSynthesis API. Provide clear error messages to users.
- Internationalization: If your application supports multiple languages, consider using localization techniques to provide translations for UI elements, ensuring a consistent experience for users around the world.
- User Feedback: Include feedback mechanisms, such as notifications or status indicators, to inform users about the progress of the speech synthesis (e.g., loading indicators or confirmation messages).
- Accessibility Considerations: Beyond basic accessibility, ensure your application meets Web Content Accessibility Guidelines (WCAG). This includes ensuring that all interactive elements are navigable via keyboard and that screen readers can properly interpret the interface.
- Security Best Practices: Implement security best practices to protect against potential vulnerabilities. This includes sanitizing user input and securing API calls to prevent misuse.
- Documentation: Provide clear documentation for users on how to use the application, including how to access different features and any limitations. This will enhance user experience and support.
- Scalability: Design your application to be scalable so that it can handle increased usage or additional features in the future without major overhauls.
- Regular Updates: Keep your application up to date with the latest web technologies and security patches to ensure ongoing compatibility and protection.
Additional Notes
By keeping these additional notes in mind, you can create a Text to Speech application that is not only effective and user-friendly but also reliable, secure, and adaptable to future needs.
Conclusion and final words
Text to Speech (TTS) is a technology that converts text into sounds that resemble human speech. It plays a vital role in improving accessibility for people with disabilities, supporting applications such as virtual assistants, language learning, GPS navigation, and media content. With the ability to adjust language, speed, pitch, and volume, TTS is a flexible solution that is becoming increasingly common in everyday life.
Despite some limitations such as voices that still sound less natural in some systems, developments in AI and Natural Language Processing (NLP) continue to improve the quality of synthetic voices. TTS plays a crucial role in accelerating access to information, making this technology a useful tool in the future.
Text to Speech technology opens a new window for accessibility and communication in the digital age. With continued development, TTS is getting closer to natural human speech and providing innovative solutions for various interaction needs with technology. In the future, we can expect improved voice quality, more emotional interactions, and smoother pronunciation, making TTS increasingly integral to modern life.
If you are having trouble creating a Text to Speech application using Bootstrap, TailwindCSS, JSON and JavaScript, you can download the source code of this project for free by clicking the “Download” button. You can also view a live demo of this application by clicking the “View Live” button to see how the TTS design and functionality work in real time.
By accessing the source code and live demo, you can easily understand the code implementation and customize the project to your needs. Feel free to explore the code, experiment with the design, and modify the features to improve your skills in using HTML, CSS, and JavaScript.
This is a great opportunity to learn more about integrating CSS frameworks with JavaScript and deepen your understanding of interactive web applications and accessibility. Happy learning and experimenting!
LEAVE A REPLY