Building a AES Encoder Decoder using HTML, CSS & JavaScript
Saturday, August 24, 2024
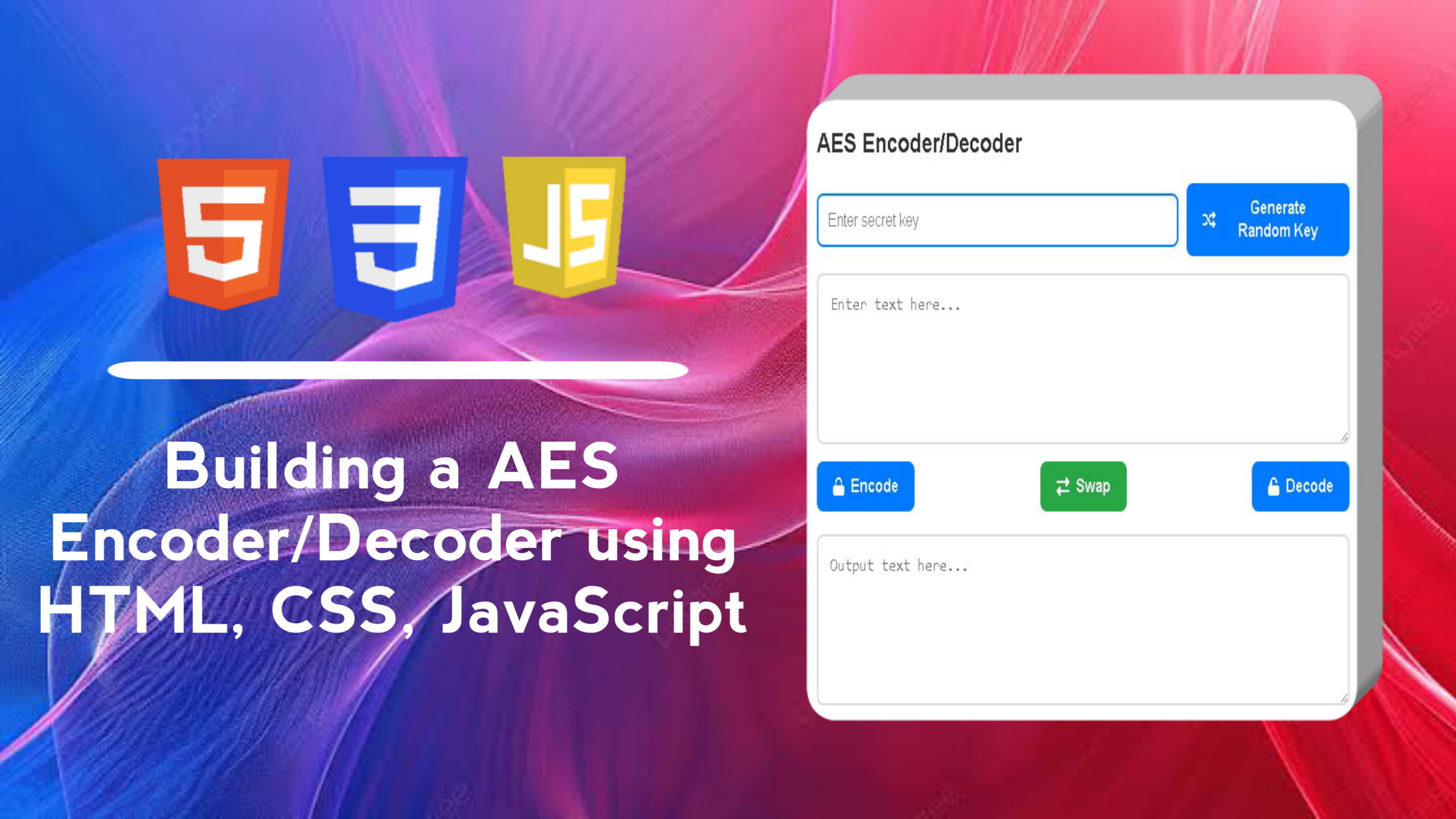
This AES Encoder/Decoder application allows users to encrypt and decrypt text using the AES (Advanced Encryption Standard) algorithm. The AES algorithm is one of the symmetric encryption standards that is widely used to maintain data security. This application is designed with a simple interface using HTML and CSS, and works with the help of the CryptoJS library in JavaScript.
In this blog post, I will walk you through creating an AES Encoder/Decoder application using HTML, CSS, and JavaScript. We will replicate the main functionality of the text encryption and decryption process using the AES (Advanced Encryption Standard) algorithm. The project includes creating an intuitive interface with input bars for text and secret keys, buttons for encrypting and decrypting, and a feature for generating random keys.
As you work on this project, you’ll learn how to structure and style websites effectively to ensure they look great on all devices.
Key Features:
- AES Encryption: Text encryption using the AES (Advanced Encryption Standard) algorithm to ensure your data remains secure and unreadable without the correct key.
- AES Decryption: Decrypts encrypted text to return the data to its original form, allowing you to access the information that has been encrypted.
- Random Key Generation: A feature to automatically generate random keys, making it easier for you to get secure keys without having to create them manually.
- Swap Input and Output: Ability to swap text between input and output areas, so you can easily check the encryption and decryption results.
- Responsive User Interface: Responsive design allows the app to function well across a variety of devices and screen sizes, providing a consistent user experience.
With these features, your AES Encoder/Decoder application will have complete functionality and an attractive design, making it not only useful but also fun to use.
Why Building a AES Encoder Decoder using HTML, CSS, JavaScript?
- Application of Encryption Algorithms: You will understand how to use AES (Advanced Encryption Standard) to encrypt and decrypt data, which is an important skill in data security.
- User Interface Design: You will learn how to create intuitive and responsive user interfaces using HTML and CSS, including layout, element styling, and design responsiveness.
- JavaScript Library Integration: You will learn how to integrate libraries like CryptoJS to handle encryption and decryption directly in the browser.
- Interactivity with JavaScript: You will gain experience writing JavaScript to add interactivity, such as buttons to activate encryption, decryption, and random key generation functions.
- Input and Output Management: You will understand how to handle user input and display results in an effective manner.
By building an AES Encoder/Decoder project using HTML, CSS and JavaScript, you will gain the following skills:
This project will provide a solid foundation in responsive web design and front-end development, paving the way for more complex web projects in the future. Good luck and may the skills you gain be useful in developing future projects!
Steps to make a AES Encoder Decoder using HTML, CSS and JavaScript
Here are the steps to create an AES Encoder/Decoder application using HTML, CSS, and JavaScript:
1. Create Basic HTML Structure
The HTML structure defines the main elements on the page such as text input, buttons for encoder/decoder, and output areas. HTML also links the CryptoJS library used to perform encryption and decryption.
<!DOCTYPE html>
<!-- Coding By CodingIndo - https://codingindo.vercel.app/ -->
<html lang="en">
<head>
<!-- Meta tag for character encoding -->
<meta charset="UTF-8">
<!-- Meta tag to make the viewport responsive on mobile devices -->
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Page title -->
<title>AES Encoder/Decoder</title>
<!-- Link to CSS file for styling -->
<link rel="stylesheet" href="style.css">
<!-- Link to Font Awesome for icons -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0-beta3/css/all.min.css">
<!-- Link to CryptoJS for encryption and decryption -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/crypto-js/3.1.9-1/crypto-js.js"></script>
</head>
<body>
<div class="container">
<!-- Application title -->
<h1>AES Encoder/Decoder</h1>
<div class="tools">
<div class="tool">
<div class="key-container">
<!-- Input to enter secret key -->
<input type="text" id="secret-key" placeholder="Enter secret key">
<!-- Button to generate random key -->
<button id="generate-key-btn"><i class="fas fa-random"></i> Generate Random Key</button>
</div>
<!-- Textarea to enter text to be encrypted or decrypted -->
<textarea id="input-text" placeholder="Enter text here..."></textarea>
<div class="button-group">
<!-- Button to encrypt text -->
<button id="encode-btn"><i class="fas fa-lock"></i> Encode</button>
<!-- Button to swap text between input and output -->
<button id="swap-btn"><i class="fas fa-exchange-alt"></i> Swap</button>
<!-- Button to decrypt text -->
<button id="decode-btn"><i class="fas fa-unlock"></i> Decode</button>
</div>
</div>
<div class="tool">
<!-- Textarea to display encrypted or decrypted text -->
<textarea id="output-text" placeholder="Output text here..."></textarea>
</div>
</div>
</div>
<!-- Link to JavaScript file for functionality -->
<script src="script.js"></script>
</body>
</html>
Explanation
Number | Line of Code | Explanation |
---|
2. Add Style with CSS
To make the application look neat, use CSS to design the layout and make it responsive.
/* Sets styles for the body element */
body {
font-family: 'Arial', sans-serif;
margin: 0;
padding: 0;
background: linear-gradient(to right, #6a11cb, #2575fc);
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
/* Sets styles for container elements */
.container {
background-color: #ffffff;
padding: 20px;
border-radius: 12px;
box-shadow: 0 8px 16px rgba(0, 0, 0, 0.2);
max-width: 700px;
width: 100%;
box-sizing: border-box;
}
/* Sets the style for the h1 element */
h1 {
color: #333;
margin-bottom: 20px;
font-size: 1.5em;
font-weight: bold;
}
/* Sets the style for the tools element */
.tools {
display: flex;
flex-direction: column;
gap: 20px;
}
/* Sets the style for the tool element */
.tool {
display: flex;
flex-direction: column;
gap: 15px;
}
/* Sets styles for key-container elements */
.key-container {
display: flex;
flex-direction: column;
gap: 10px;
align-items: stretch;
}
/* Sets the style for a text input element */
input[type="text"] {
width: 100%;
padding: 12px;
border: 2px solid #007bff;
border-radius: 8px;
font-size: 16px;
transition: border-color 0.3s ease;
}
/* Sets the style for a text input element when focused */
input[type="text"]:focus {
border-color: #0056b3;
outline: none;
}
/* Sets the style for a textarea element */
textarea {
width: 100%;
height: 150px;
padding: 15px;
border: 2px solid #ddd;
border-radius: 8px;
font-size: 16px;
box-sizing: border-box;
transition: border-color 0.3s ease;
}
/* Sets the style for a textarea element when it is focused */
textarea:focus {
border-color: #007bff;
outline: none;
}
/* Sets the style for the button element */
button {
padding: 12px 20px;
border: none;
border-radius: 8px;
background-color: #007bff;
color: #fff;
cursor: pointer;
font-size: 16px;
font-weight: bold;
transition: background-color 0.3s ease, transform 0.2s ease;
display: flex;
align-items: center;
gap: 8px;
}
/* Sets the style for a button element on hover */
button:hover {
background-color: #0056b3;
}
/* Sets the style for the button element when active */
button:active {
transform: scale(0.98);
}
/* Sets the style for the button element with id swap-btn */
#swap-btn {
background-color: #28a745;
}
/* Sets the style for the button element with id swap-btn on hover */
#swap-btn:hover {
background-color: #218838;
}
/* Sets the style for the button-group element */
.button-group {
display: flex;
flex-direction: column;
gap: 10px;
}
/* Sets the style for the button elements inside the button-group */
.button-group button {
width: 100%;
}
/* Sets styles for elements in media queries with a minimum width of 768px */
@media (min-width: 768px) {
/* Sets styles for key-container elements */
.key-container {
flex-direction: row;
align-items: center;
}
/* Sets the style for a text input element */
input[type="text"] {
width: calc(100% - 150px);
}
/* Sets the style for the button-group element */
.button-group {
flex-direction: row;
justify-content: space-between;
}
/* Sets the style for the button elements inside the button-group */
.button-group button {
width: auto;
}
}
Explanation
Number | Line of Code | Explanation |
---|
3. Add Logic with JavaScript
Use JavaScript to make the application work. Here is the code for encoding and decoding using the CryptoJS library.
// Event listener for encode button
document.getElementById('encode-btn').addEventListener('click', function() {
var text = document.getElementById('input-text').value;
var key = document.getElementById('secret-key').value;
var encrypted = CryptoJS.AES.encrypt(text, key).toString();
document.getElementById('output-text').value = encrypted;
});
// Event listener for decode button
document.getElementById('decode-btn').addEventListener('click', function() {
var encryptedText = document.getElementById('input-text').value;
var key = document.getElementById('secret-key').value;
var decrypted = CryptoJS.AES.decrypt(encryptedText, key).toString(CryptoJS.enc.Utf8);
document.getElementById('output-text').value = decrypted;
});
// Event listener for swap button
document.getElementById('swap-btn').addEventListener('click', function() {
var inputText = document.getElementById('input-text').value;
var outputText = document.getElementById('output-text').value;
document.getElementById('input-text').value = outputText;
document.getElementById('output-text').value = inputText;
});
// Event listener for generate key button
document.getElementById('generate-key-btn').addEventListener('click', function() {
var randomKey = CryptoJS.lib.WordArray.random(16).toString(CryptoJS.enc.Hex);
document.getElementById('secret-key').value = randomKey;
});
Explanation
Number | Line of Code | Explanation |
---|
- Generate a random key.
- Enter text and encode it.
- Decode the encoded text.
- Swap the text between input and output areas.
4. Run Your Project and Customize
Open the HTML file in a browser and test the functionalities:
- Key Security: Make sure to use strong and secure keys when encrypting data. Weak keys can make the encryption easier to hack.
- Input Validation: It is recommended to add input validation to ensure that the text and key are not empty before encrypting or decrypting, to prevent user errors.
- Sensitive Data Protection: Encrypted data and secret keys should not be stored carelessly, especially if the project is used in an environment that requires high security.
- Browser Compatibility: Check the application's compatibility with various browsers to ensure that all functions, especially those related to JavaScript and encryption, work properly across platforms.
- UX/UI improvements: You can add additional interface elements such as notifications or loading indicators to improve the user experience during the encryption or decryption process.
Additional Notes
By keeping these things in mind, your AES Encoder/Decoder application will be more secure, easier to use, and have a better user experience.
Conclusion and final words
By following the steps above, you have successfully created a simple AES Encoder/Decoder application using HTML, CSS, and JavaScript. This application utilizes the AES (Advanced Encryption Standard) algorithm which is one of the most secure encryption methods and is often used in various data security systems. You have combined the CryptoJS library to perform encryption and decryption directly in the browser without the need for a backend or server.
Some key points of this project:
- You learned how to use HTML to create the basic structure of an application.
- You design the appearance of the application with CSS, so that the application looks attractive and responsive.
- You integrate JavaScript and the CryptoJS library to perform encryption and decryption with the AES algorithm.
- You also add interactive features like generating random keys and swapping input and output text.
Good luck, and keep experimenting with different web technologies to create more innovative solutions! 😊
If you are having trouble creating an AES Encoder/Decoder application using HTML, CSS, and JavaScript, you can download the source code of this project for free by clicking the “Download” button. You can also view a live demo of this application by clicking the “View Live” button to see how the design and functionality of encryption and decryption work in practice.
By accessing the source code and live demo, you can easily understand the code implementation and customize the project according to your needs. Feel free to explore the code, experiment with styles, and modify features to improve your skills in web development and data encryption.
This is a great opportunity to learn more about encryption with AES and improve your understanding of the integration between HTML, CSS, and JavaScript. Happy learning and experimenting!
LEAVE A REPLY