Heatmap Color in HTML, CSS & JavaScript
Sunday, August 29, 2024
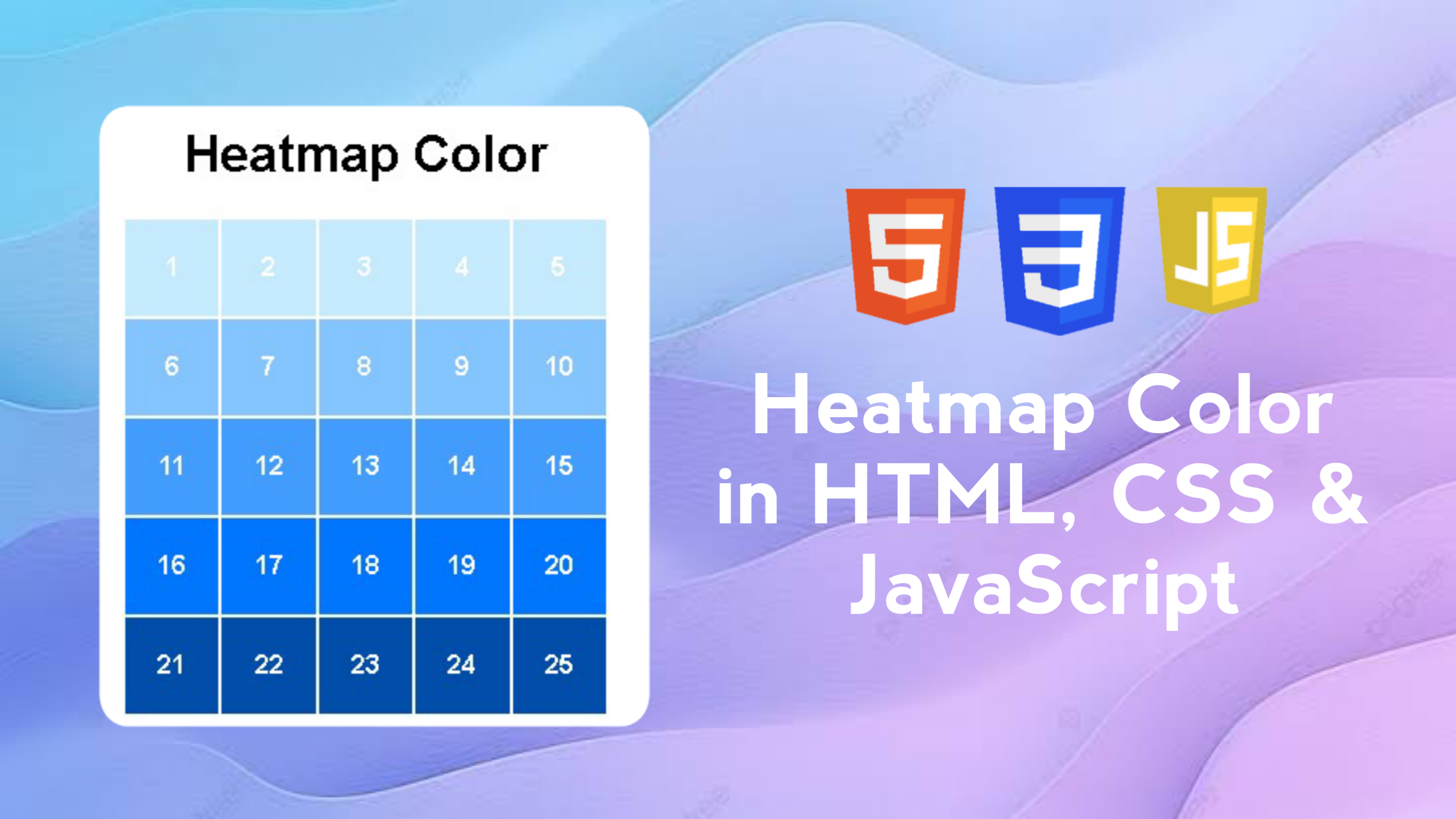
A Heatmap Color is a visual representation of data where individual values are represented by colors. In this tutorial, we will create a simple heatmap consisting of a grid (table) where each cell is colored based on a specific numeric value. We will use HTML for the structure, CSS for styling, and JavaScript for logic and data manipulation.
In this blog post, I will walk you through creating a simple heatmap using HTML, CSS, and JavaScript. We will create a heatmap view that visualizes numeric data using color to indicate intensity or value. The heatmap we will create includes key features such as:
- Navigation Bar: Sets the main view of the page with titles.
- Grid Layout: Use CSS Grid to neatly arrange the heatmap boxes.
- Dynamic Coloring: Applying different colors based on numeric values to provide clear visualization.
- Responsiveness: Ensures the heatmap displays well on various screen sizes.
As you work on this project, you’ll learn how to structure and style websites effectively to ensure they look great on all devices.
Key Features:
- HTML Structure: Lays out the basic elements for the heatmap, including the main container that holds all the data boxes.
- Grid Layout with CSS: Use CSS Grid to neatly layout the heatmap boxes in a grid format.
- Dynamic Coloring: Apply different colors to each box based on numeric values using JavaScript, to provide clear and informative visualizations.
- Responsiveness: Adjust the heatmap display to maintain proper proportions across screen sizes, using CSS properties such as max-width and margin.
- DOM Manipulation with JavaScript: Dynamically create and add div elements to the heatmap container, and set styles and content based on data.
- Data Visualization: Using colors to represent data values, facilitating visual understanding and analysis of data.
With these features, you will have effective and attractive heatmaps, as well as improve your skills in using HTML, CSS, and JavaScript to build data visualizations.
Why make a heatmap color in HTML, CSS & JavaScript?
- HTML & Data Structures: Understand how to structure HTML elements to create the basic structure of a heatmap.
- CSS Grid Layout: Master the CSS Grid technique to arrange heatmap boxes in a neat and responsive grid layout.
- Dynamic Coloring with CSS: Learn how to apply dynamic, responsive styling to grid elements to reflect data values.
- DOM Manipulation with JavaScript: Develop skills in using JavaScript to dynamically create elements, style them, and add data to heatmaps.
- Data Visualization: Improve skills in creating effective data visualizations through the use of color to indicate intensity or value in a heatmap.
By building this heatmap project using HTML, CSS, and JavaScript, you will gain the following skills:
This project will provide a solid foundation in responsive web design and front-end development, paving the way for more complex web projects in the future. Good luck and may the skills you gain be useful in developing future projects!
Steps to make a heatmap color using HTML, CSS & JavaScript
- Create a div element with the id heatmap as the container of the heatmap.
- Add an h1 element as a title.
Here are the steps to create a Color Heatmap using HTML, CSS, and JavaScript:
1. Create HTML Structure
Create an HTML file as the basic structure of the heatmap. Use div elements to contain the heatmap boxes that will later be filled by JavaScript.
<!DOCTYPE html>
<!-- Coding By CodingIndo - https://codingindo.vercel.app/ -->
<html lang="en">
<head>
<!-- Meta tag for character encoding -->
<meta charset="UTF-8">
<!-- Meta tag to make the viewport responsive on mobile devices -->
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Page title -->
<title>Heatmap Color</title>
<!-- Link to CSS file for styling -->
<link rel="stylesheet" href="style.css">
</head>
<body>
<!-- Page title with centered text -->
<h1 style="text-align: center;">Heatmap Color</h1>
<!-- Div to display heatmap color -->
<div id="heatmap" class="heatmap"></div>
<!-- Link to JavaScript file for functionality -->
<script src="script.js"></script>
</body>
</html>
Explanation
Number | Line of Code | Explanation |
---|---|---|
1 | <!DOCTYPE html> | The document type declaration used is HTML5. |
2 | <!-- Coding By CodingIndo - https://codingindo.vercel.app/ --> | Comments stating that this code was created by CodingIndo, and listing their website URL. |
3 | <html lang="en"> | The opening tag for the <html> element with the lang="en" attribute indicates that the language of this document is English. |
4 | <head> | The opening tag for the <head> element, which contains metadata and settings for the document. |
5 | <!-- Meta tag for character encoding --> | A comment explaining that the following line is a meta tag for character encoding. |
6 | <meta charset="UTF-8"> | Meta tag to set the character encoding to UTF-8, so that the document supports various characters and symbols. |
7 | <!-- Meta tag to make the viewport responsive on mobile devices --> | The comment indicates that the following line is to adjust the display on mobile devices. |
8 | <meta name="viewport" content="width=device-width, initial-scale=1.0"> | Meta tag to create a responsive document display on mobile devices, with a display width according to the device and an initial scale of 1. |
9 | <!-- Page title --> | A comment explaining that the following line will contain the page title. |
10 | <title>Heatmap Color</title> | The <title> tag displays the title of the "Heatmap Color" page on the browser tab. |
11 | <!-- Link to CSS file for styling --> | A comment indicating that the following line is a link to an external CSS file for styling. |
12 | <link rel="stylesheet" href="style.css"> | The <link> tag links to an external CSS file style.css to apply styles to the page. |
13 | </head> | The closing tag for the <head> element. |
14 | <body> | The opening tag for the <body> element, which contains the content that will be displayed on the web page. |
15 | <!-- Page title with centered text --> | A comment indicating that the following line is the page title with centered text. |
16 | <h1 style="text-align: center;">Heatmap Color</h1> | The <h1> tag displays the title "Heatmap Color" with centered text. |
17 | <!-- Div to display heatmap color --> | The comment indicates that the next element is a <div> to display the heatmap colors. |
18 | <div id="heatmap" class="heatmap"></div> | The <div> tag with the attributes id="heatmap" and class="heatmap" to display a heatmap on a web page. |
19 | <!-- Link to JavaScript file for functionality --> | A comment explaining that the following line is a link to an external JavaScript file to add functionality. |
20 | <script src="script.js"></script> | The <script> tag links to an external JavaScript file script.js to add heatmap functionality to a web page. |
21 | </body> | The closing tag for the <body> element. |
22 | </html> | The closing tag for the <html> element. |
- Use CSS Grid to create a grid-like layout.
- Set the color and size of the box and the text inside the box.
2. Add CSS for Styling
Add styling using CSS to create a grid on the heatmap and adjust its appearance.
/* Sets the font for the entire body */
body {
font-family: Arial, sans-serif;
}
/* Sets the grid display for the heatmap */
.heatmap {
display: grid;
grid-template-columns: repeat(5, 1fr);
gap: 2px;
max-width: 300px;
margin: 0 auto;
}
/* Sets the appearance of each div in the heatmap */
.heatmap div {
padding: 20px;
text-align: center;
color: white;
font-weight: bold;
}
Explanation
Number | Line of Code | Explanation |
---|---|---|
1 | /* Sets the font for the entire body */ | A comment explaining that the following section will set the font for all elements inside <body>. |
2 | body { | Opens the style declaration for the <body> element. |
3 | font-family: Arial, sans-serif; | Sets the font for the entire page to use "Arial" as the primary font. If Arial is not available, another sans-serif font will be used. |
4 | } | Closes the style declaration for the <body> element. |
5 | /* Sets the grid display for the heatmap */ | The comment explains that the following section will set the grid display for elements with the .heatmap class. |
6 | .heatmap { | Opens the style declaration for an element with the .heatmap class. |
7 | display: grid; | Sets the .heatmap element to use grid view, enabling a grid-based layout for the items within it. |
8 | grid-template-columns: repeat(5, 1fr); | Defines 5 columns in the grid, each taking up 1 equal fraction of the width of the .heatmap container. |
9 | gap: 2px; | Adds a 2 pixel spacing between each element in the grid, creating a small separation between elements in the heatmap. |
10 | max-width: 300px; | Limits the maximum width of the .heatmap container to 300 pixels, so that the heatmap does not expand beyond this limit. |
11 | margin: 0 auto; | Set the top and bottom margins to 0 and the left and right margins to auto, to make the .heatmap container horizontally centered on the page. |
12 | } | Closes the style declaration for an element with the .heatmap class. |
13 | /* Sets the appearance of each div in the heatmap */ | The comment explains that the following section will control the appearance of each <div> element within the .heatmap. |
14 | .heatmap div { | Opens the style declaration for each <div> element inside the .heatmap container. |
15 | padding: 20px; | Gives 20 pixels of padding inside each <div> element, thus providing space inside the element. |
16 | text-align: center; | Set the text inside each <div> to center align. |
17 | color: white; | Sets the text color to white to increase contrast on the heatmap's usually darker background. |
18 | font-weight: bold; | Sets the text inside each <div> to bold. |
19 | } | Closes the style declaration for each <div> element inside .heatmap. |
- Create a 2D array containing numeric data.
- Create a function getColor() that determines the color based on a numeric value.
- Loop the data to create boxes with the appropriate values and colors.
3. Add JavaScript to Create Heatmap
Use JavaScript to add box elements to the heatmap based on the given data values.
// Get element with id 'heatmap'
const heatmapContainer = document.getElementById('heatmap');
// Data for heatmap
const data = [
[1, 2, 3, 4, 5],
[6, 7, 8, 9, 10],
[11, 12, 13, 14, 15],
[16, 17, 18, 19, 20],
[21, 22, 23, 24, 25]
];
// Function to get color based on value
function getColor(value) {
if (value <= 5) return 'rgb(198, 235, 255)';
if (value <= 10) return 'rgb(132, 196, 255)';
if (value <= 15) return 'rgb(66, 157, 255)';
if (value <= 20) return 'rgb(0, 118, 255)';
return 'rgb(0, 78, 170)';
}
// Iterate through each row of data
data.forEach(row => {
// Iterate through each value in the row
row.forEach(value => {
// Create a div element for each value
const cell = document.createElement('div');
// Sets the background color based on the value
cell.style.backgroundColor = getColor(value);
// Add value text into div element
cell.textContent = value;
// Add a div element to the heatmap container
heatmapContainer.appendChild(cell);
});
});
Explanation
Number | Line of Code | Explanation |
---|---|---|
1 | // Get element with id 'heatmap' | The comment indicates that the following line will fetch the element with the id 'heatmap'. |
2 | const heatmapContainer = document.getElementById('heatmap'); | Declare a variable heatmapContainer that stores the element with id="heatmap" from the DOM, which will be the container for the heatmap. |
3 | // Data for heatmap | A comment indicating that the following line will define the data for the heatmap. |
4 | const data = [[1, 2, 3, 4, 5], [6, 7, 8, 9, 10], [11, 12, 13, 14, 15], [16, 17, 18, 19, 20], [21, 22, 23, 24, 25]]; | Declares a data variable containing a 2D array, where each element represents a value in a heatmap grid (5x5). |
5 | // Function to get color based on value | A comment indicating that the following line will define a function to determine the color based on a given value. |
6 | function getColor(value) { | Defines a function getColor that accepts a value parameter and returns a color based on that value. |
7 | if (value <= 5) return 'rgb(198, 235, 255)'; | If the value is less than or equal to 5, the function returns the color rgb(198, 235, 255), which is a light blue color. |
8 | if (value <= 10) return 'rgb(132, 196, 255)'; | If the value is less than or equal to 10, the function returns the color rgb(132, 196, 255), which is darker than the previous color. |
9 | if (value <= 15) return 'rgb(66, 157, 255)'; | If the value is less than or equal to 15, the function returns the color rgb(66, 157, 255), which is even darker. |
10 | if (value <= 20) return 'rgb(0, 118, 255)'; | If the value is less than or equal to 20, the function returns the color rgb(0, 118, 255), which is a darker blue. |
11 | return 'rgb(0, 78, 170)'; | If the value is greater than 20, the function returns the color rgb(0, 78, 170), which is the darkest blue in this scale. |
12 | } | Closing the getColor function declaration. |
13 | // Iterate through each row of data | A comment indicating that the following line will iterate over each row in the heatmap data. |
14 | data.forEach(row => { | Using the forEach method to iterate over each row in the data array. |
15 | // Iterate through each value in the row | A comment indicating that the following line will iterate over each value in the row. |
16 | row.forEach(value => { | Using the forEach method to iterate over each value in a row. |
17 | // Create a div element for each value | A comment indicating that a <div> element will be created for each value in the grid. |
18 | const cell = document.createElement('div'); | Declares a cell variable that creates a new <div> element to hold the values from the heatmap. |
19 | // Sets the background color based on the value | The comment indicates that the following line will set the background color based on the value. |
20 | cell.style.backgroundColor = getColor(value); | Sets the background color of a cell element by calling the getColor function to determine the appropriate color based on the value. |
21 | // Add value text into div element | A comment indicating that the value text will be added to the <div> element. |
22 | cell.textContent = value; | Inserts value text into cell elements, so that each cell shows the appropriate value. |
23 | // Add a div element to the heatmap container | A comment indicating that a cell element will be added to the heatmap container. |
24 | heatmapContainer.appendChild(cell); | Adds a cell element to the heatmapContainer element, so that it appears on the page as part of the heatmap. |
25 | }); | Closes the second forEach, which iterates over the values within each row. |
26 | }); | Closes the first forEach, which iterates over each row in the heatmap data. |
4. Run Your Project
Once all the code is ready, save the file with the .html extension. For example, save it as heatmap.html. Open the file in a browser to see the heatmap result.
The final result will display a 5x5 grid with each box a different color based on the value inside it.
- Data Readability:
- Make sure that the colors used in the heatmap contrast sufficiently with the text to ensure good readability.
- Consider providing a legend or color guide to help users understand the meaning of the various colors.
- Responsiveness:
- Test the heatmap display on different devices and screen sizes to ensure that the layout and box sizes remain proportional and easy to read.
- Use media queries if necessary to adjust CSS styles on devices with different screen sizes.
- Performance Optimization: If the data being displayed is very large, consider optimizing performance by reducing the number of DOM elements created or using lazy loading techniques.
- Accessibility: Add ARIA attributes or alternative text if necessary to ensure that the heatmap is accessible to users with special accessibility needs.
- Testing: Perform thorough testing on the heatmap to ensure that all functions are working properly and there are no bugs that interfere with the user experience.
Additional Notes
By paying attention to these additional points, you can improve the quality and usability of your heatmap, ensuring that it is not only functionally effective but also user-friendly and accessible.
Conclusion and final words
Creating a simple heatmap color using HTML, CSS and JavaScript is a process that involves a few basic steps:
- HTML Structure: Provides a framework for displaying heatmaps with div elements as containers.
- CSS Styling: Sets the layout and style of the heatmap, such as grid size, box spacing, and text styling.
- JavaScript Manipulation: Populate the heatmap with dynamic data, set the background color of the box based on the data value, and add the box element to the container.
Creating heatmap color is an effective way to present numerical data in an intuitive and easy-to-understand way. With a combination of HTML, CSS and JavaScript, you can build compelling and useful data visualizations for a variety of applications, whether in data analysis, dashboards or information presentations.
Keep experimenting with different data and designs to improve your skills in creating effective data visualizations. If you have further questions or need help, don't hesitate to ask!
Good luck, and may your heatmap project be a success! 🚀
If you are having trouble creating a color heatmap using HTML, CSS and JavaScript, you can download the source code file of this project for free by clicking the "Download" button. You can also view a live demo of this color heatmap by clicking the "View Live" button to see how the design and functionality of the color heatmap work in practice.
By accessing the source code file and live demo, you can easily understand the code implementation and customize the project according to your needs. Feel free to explore the code, experiment with dynamic coloring, and modify the features to improve your skills in web development.
This is a great opportunity to learn more about the integration of HTML, CSS Grid, and JavaScript to create interactive data visualizations. Happy learning and experimenting!
LEAVE A REPLY