How to make a currency converter using Bootstrap, TailwindCSS & JavaScript
Sunday, August 11, 2024
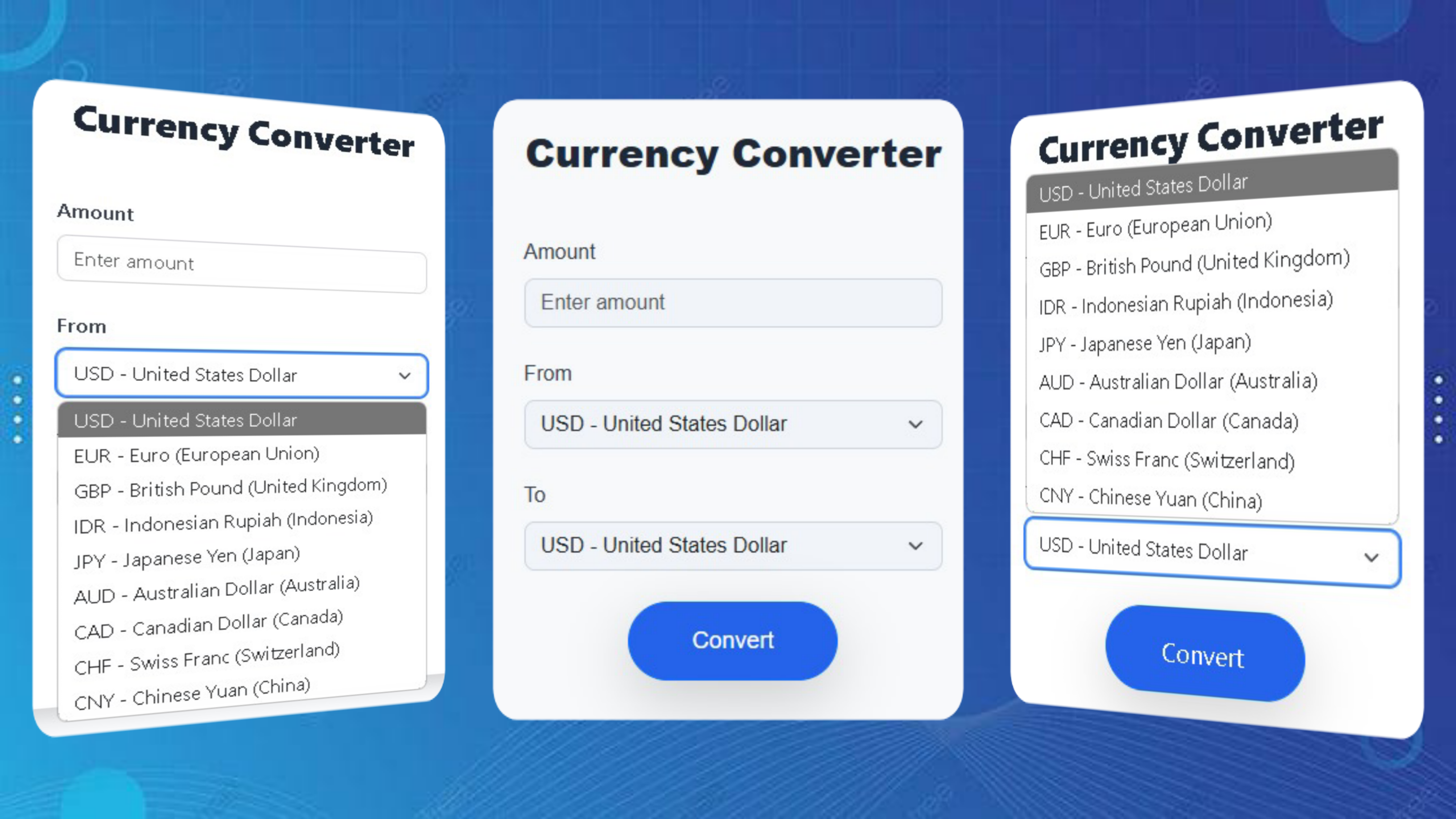
This project aims to create a simple Currency Converter application that allows users to convert amounts of money from one currency to another. The application uses Bootstrap 5 for basic styling, TailwindCSS for additional styling, and JavaScript for the currency conversion logic using an external API.
In this blog post, I’ll walk you through creating a responsive currency converter app using Bootstrap, TailwindCSS, and JavaScript. We’ll replicate the core features of a currency converter app, including inputting amounts and currency selections, displaying conversion results, and integrating APIs to get up-to-date currency rates. Additionally, we’ll make sure the app is responsive and easy to use across devices, while maintaining a modern design with customization options using TailwindCSS.
As you work on this project, you’ll learn how to structure and style websites effectively to ensure they look great on all devices.
Key Features:
- Input Amount and Currency Selection: Users can enter the amount and select the source and destination currencies from the dropdown list.
- Real-Time Currency Conversion: The app uses external APIs to calculate current currency rates and displays conversion results in real time.
- Responsive and Flexible: With Bootstrap, the app automatically adjusts the layout for different screen sizes, ensuring an optimal user experience on both mobile and desktop devices.
- Design Customization with TailwindCSS: The app uses TailwindCSS to provide flexibility in design customization, allowing developers to easily add styles as needed.
- Simple and User-Friendly Navigation: Simple interface with intuitive navigation elements, so users can easily enter data and get conversion results.
With these features, your currency converter will have complete functionality and an attractive design, making it not only useful but also enjoyable to use.
Why make a currency converter in Bootstrap, TailwindCSS & JavaScript?
- Bootstrap: Learn how to use Bootstrap components and grid system to quickly build responsive layouts.
- TailwindCSS: Master utility-first CSS techniques to customize designs without writing a lot of additional CSS code.
- JavaScript: Learn how to create dynamic applications with JavaScript, including fetching data from external APIs to perform real-time currency conversions.
- Responsive App Development: Understand how to combine Bootstrap and Tailwind to create responsive and optimized user experiences across devices.
- API Interaction: Integrate external APIs to get currency rate data and dynamically update the interface based on user input.
By building this currency converter project using Bootstrap, TailwindCSS, and JavaScript, you can gain the following skills:
This project will provide a solid foundation in responsive web design and front-end development, paving the way for more complex web projects in the future. Good luck and may the skills you gain be useful in developing future projects!
Steps to make a currency converter using Bootstrap, TailwindCSS & JavaScript
- Create Project Folder: Create a new folder for your project, for example currency-converter.
- Create HTML File: Inside the project folder, create an HTML file, for example index.html.
- Create a JavaScript File: Inside the same project folder, create a JavaScript file, for example script.js.
- Create a CSS File (Optional): If you want to add additional CSS, create a CSS file, for example styles.css.
Here are the detailed steps to create a currency converter using Bootstrap, TailwindCSS, and JavaScript:
1. Prepare Project Structure
2. Write HTML Code
Add the following HTML code to index.html.
<!DOCTYPE html>
<!-- Coding By CodingIndo - https://codingindo.vercel.app/ -->
<html lang="en">
<head>
<!-- Meta tag for character encoding -->
<meta charset="UTF-8">
<!-- Meta tag for setting the viewport to be responsive on mobile devices -->
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Page title -->
<title>Currency Converter</title>
<!-- Link to CSS file for styling -->
<link rel="stylesheet" href="style.css">
<!-- Bootstrap CSS link for styling -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
<!-- TailwindCSS link for styling -->
<link href="https://cdn.jsdelivr.net/npm/tailwindcss@2.2.19/dist/tailwind.min.css" rel="stylesheet">
</head>
<body class="bg-gray-50">
<div class="container mt-5">
<div class="card shadow p-5 rounded-xl border-0">
<!-- Application title -->
<h2 class="text-center mb-5 text-3xl font-extrabold text-gray-800">Currency Converter</h2>
<!-- Form for currency conversion -->
<form id="converter-form">
<div class="mb-4">
<!-- Labels and input for money amounts -->
<label for="amount" class="form-label text-gray-700 font-medium">Amount</label>
<input type="number" class="form-control rounded-lg border-gray-300 focus:ring-2 focus:ring-blue-500" id="amount" placeholder="Enter amount" required>
</div>
<div class="mb-4">
<!-- Label and select for the source currency -->
<label for="from-currency" class="form-label text-gray-700 font-medium">From</label>
<select class="form-select rounded-lg border-gray-300 focus:ring-2 focus:ring-blue-500" id="from-currency">
<option value="USD" selected>USD - United States Dollar</option>
<option value="EUR">EUR - Euro (European Union)</option>
<option value="GBP">GBP - British Pound (United Kingdom)</option>
<option value="IDR">IDR - Indonesian Rupiah (Indonesia)</option>
<option value="JPY">JPY - Japanese Yen (Japan)</option>
<option value="AUD">AUD - Australian Dollar (Australia)</option>
<option value="CAD">CAD - Canadian Dollar (Canada)</option>
<option value="CHF">CHF - Swiss Franc (Switzerland)</option>
<option value="CNY">CNY - Chinese Yuan (China)</option>
</select>
</div>
<div class="mb-4">
<!-- Label and select for destination currency -->
<label for="to-currency" class="form-label text-gray-700 font-medium">To</label>
<select class="form-select rounded-lg border-gray-300 focus:ring-2 focus:ring-blue-500" id="to-currency">
<option value="USD">USD - United States Dollar</option>
<option value="EUR">EUR - Euro (European Union)</option>
<option value="GBP">GBP - British Pound (United Kingdom)</option>
<option value="IDR">IDR - Indonesian Rupiah (Indonesia)</option>
<option value="JPY">JPY - Japanese Yen (Japan)</option>
<option value="AUD">AUD - Australian Dollar (Australia)</option>
<option value="CAD">CAD - Canadian Dollar (Canada)</option>
<option value="CHF">CHF - Swiss Franc (Switzerland)</option>
<option value="CNY">CNY - Chinese Yuan (China)</option>
</select>
</div>
<div class="text-center">
<!-- Button to perform conversion -->
<button type="submit" class="btn btn-primary btn-lg px-5 py-3 shadow-lg hover:shadow-xl rounded-full bg-blue-600 hover:bg-blue-700 transition-transform transform hover:-translate-y-1">Convert</button>
</div>
</form>
<!-- Div to display conversion results -->
<div id="result" class="text-center mt-4 text-gray-800 text-xl font-bold"></div>
</div>
</div>
<!-- JavaScript file link for functionality -->
<script src="script.js"></script>
<!-- Bootstrap JavaScript link for functionality -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
Explanation
Number | Line of Code | Explanation |
---|
3. Add Additional Styling (Optional)
If you want to add additional styling with Tailwind CSS, you can add Tailwind CSS classes to HTML elements in index.html. You can also add custom styling in styles.css if needed.
/* Sets the font for the body element */
body {
font-family: 'Inter', sans-serif;
}
/* Sets the background and border radius for elements with class card */
.card {
background-color: #f8f9fa;
border-radius: 1rem;
}
/* Set the background, border, and transition for elements with the form-control and form-select classes */
.form-control,
.form-select {
background-color: #f1f5f9;
border: 1px solid #cbd5e1;
transition: border-color 0.3s ease, box-shadow 0.3s ease;
}
/* Sets the border and box-shadow when an element with the class form-control and form-select is in focus */
.form-control:focus,
.form-select:focus {
border-color: #6366f1;
box-shadow: 0 0 0 0.2rem rgba(99, 102, 241, 0.25);
}
/* Sets the font size for elements with class btn */
.btn {
font-size: 1.125rem;
}
/* Sets the maximum width and margin for the element with the id converter-form */
#converter-form {
max-width: 500px;
margin: 0 auto;
}
Explanation
Number | Line of Code | Explanation |
---|
4. Write JavaScript Code
Add the following JavaScript code to "script.js":
document.getElementById('converter-form').addEventListener('submit', function(e) {
e.preventDefault(); // Prevent form from default submit
const amount = document.getElementById('amount').value; // Get the amount value from input
const fromCurrency = document.getElementById('from-currency').value; // Get the source currency value from the input
const toCurrency = document.getElementById('to-currency').value; // Get the target currency value from the input
// Store the currency exchange rate in an object
const exchangeRates = {
USD: { USD: 1, EUR: 0.85, GBP: 0.75, IDR: 14500, JPY: 110, AUD: 1.35, CAD: 1.25, CHF: 0.92, CNY: 6.45 },
EUR: { USD: 1.18, EUR: 1, GBP: 0.88, IDR: 17000, JPY: 129, AUD: 1.59, CAD: 1.47, CHF: 1.08, CNY: 7.58 },
GBP: { USD: 1.34, EUR: 1.14, GBP: 1, IDR: 19500, JPY: 146, AUD: 1.81, CAD: 1.67, CHF: 1.22, CNY: 8.62 },
IDR: { USD: 0.000069, EUR: 0.000059, GBP: 0.000051, IDR: 1, JPY: 0.0075, AUD: 0.000077, CAD: 0.000073, CHF: 0.000062, CNY: 0.00053 },
JPY: { USD: 0.0091, EUR: 0.0077, GBP: 0.0068, IDR: 133.33, JPY: 1, AUD: 0.012, CAD: 0.011, CHF: 0.0084, CNY: 0.059 },
AUD: { USD: 0.74, EUR: 0.63, GBP: 0.55, IDR: 10667, JPY: 83.33, AUD: 1, CAD: 0.93, CHF: 0.69, CNY: 4.8 },
CAD: { USD: 0.8, EUR: 0.68, GBP: 0.6, IDR: 11467, JPY: 90.91, AUD: 1.08, CAD: 1, CHF: 0.74, CNY: 5.16 },
CHF: { USD: 1.09, EUR: 0.92, GBP: 0.82, IDR: 15417, JPY: 119.05, AUD: 1.45, CAD: 1.36, CHF: 1, CNY: 6.97 },
CNY: { USD: 0.16, EUR: 0.13, GBP: 0.12, IDR: 1546.15, JPY: 16.95, AUD: 0.21, CAD: 0.19, CHF: 0.14, CNY: 1 },
};
// Calculate currency conversion results
const result = (amount * exchangeRates[fromCurrency][toCurrency]).toFixed(2);
// Display the conversion result into an element with the id 'result'
document.getElementById('result').innerHTML = `
${amount} ${fromCurrency} = ${result} ${toCurrency}
`;
});
Explanation
Number | Line of Code | Explanation |
---|
- Open index.html in Browser: You can open the HTML file in a browser to see how the currency converter looks like.
- Enter Data: Try entering the amount, select the source and destination currencies, then click the "Convert" button to see the conversion results.
5. Run Your Project
- Currency Rate API: This code uses the ExchangeRate API to get exchange rate data. You may need to sign up for an API key if you use another service.
- Handling Error: Make sure to properly handle possible errors, such as no internet connection or invalid data.
Additional Notes
By paying attention to these additional notes, you can ensure that your currency converter not only works well but is also user-friendly, accessible, and secure.
Conclusion and final words
Creating a currency converter using Bootstrap, TailwindCSS, and JavaScript is an effective and efficient approach to building a functional and responsive application. Bootstrap provides pre-built UI components that speed up development, TailwindCSS provides the flexibility to customize the design without having to manually write CSS, and JavaScript acts as the engine that powers the currency conversion logic and API integration. The combination of these three technologies helps you build a modern-looking and high-performance application quickly.
By following these steps, you can create a responsive, flexible, and easy-to-maintain currency converter application. This application not only provides an intuitive user experience but also allows you to extend its functionality in the future. The combination of Bootstrap, TailwindCSS, and JavaScript is a great choice for creating a fast, elegant, and interactive web application.
If you want to add more features, such as saving conversion results or integrating more currencies, this project provides a solid foundation to build on. Happy coding!
If you are having trouble creating a currency converter using Bootstrap, TailwindCSS, and JavaScript, you can download the source code of this project for free by clicking the “Download” button. You can also view a live demo of this currency converter by clicking the “View Live” button to see how the design and functionality of the application work in practice.
By accessing the source code and live demo, you can easily understand the code implementation and customize the project according to your needs. Feel free to explore the code, experiment with styling, and modify features to improve your skills in HTML, CSS, and JavaScript.
This is a great opportunity to learn more about how to integrate CSS frameworks with JavaScript and deepen your understanding of responsive web design. Happy learning and experimenting!
LEAVE A REPLY